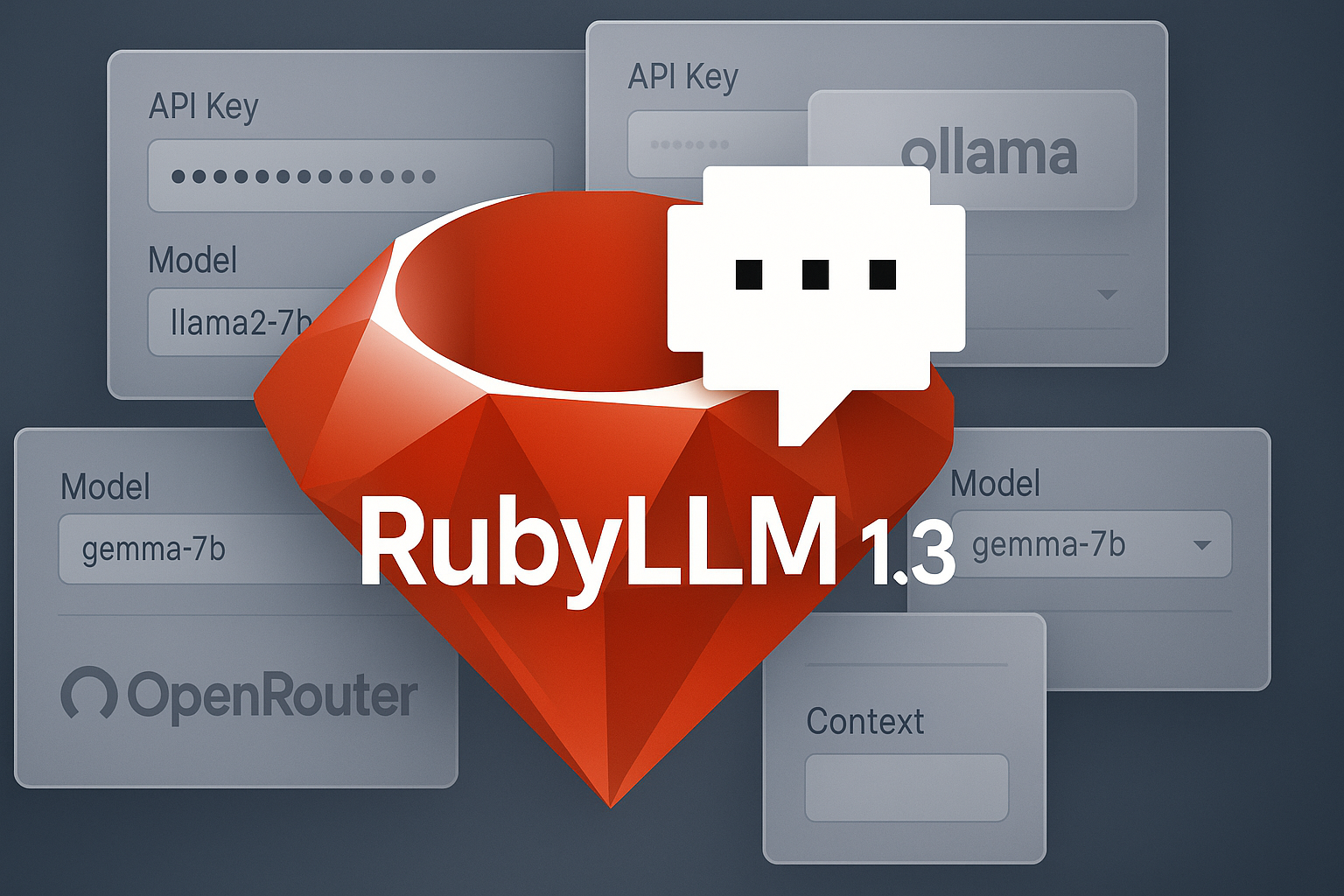
Update: This post has been updated for the stable 1.3.0 release, which shipped with all the features described below plus additional improvements from our amazing community.
RubyLLM 1.3.0 is here, and just when you thought the developer experience couldn’t get any better, we’ve made attachments ridiculously simple, added isolated configuration contexts, and officially ended the era of manual model tracking.
The Attachment Revolution: From Complex to Magical
The biggest transformation in 1.3.0 is how stupidly simple attachments have become. Before, you had to categorize every file:
# The old way (still works, but why would you?)
chat.ask "What's in this image?", with: { image: "diagram.png" }
chat.ask "Describe this meeting", with: { audio: "meeting.wav" }
chat.ask "Summarize this document", with: { pdf: "contract.pdf" }
Now? Just throw files at it and RubyLLM figures out the rest:
# The new way - pure magic ✨
chat.ask "What's in this file?", with: "diagram.png"
chat.ask "Describe this meeting", with: "meeting.wav"
chat.ask "Summarize this document", with: "contract.pdf"
# Multiple files? Mix and match without thinking
chat.ask "Analyze these files", with: [
"quarterly_report.pdf",
"sales_chart.jpg",
"customer_interview.wav",
"meeting_notes.txt"
]
# URLs work too
chat.ask "What's in this image?", with: "https://example.com/chart.png"
This is what the Ruby way looks like: you shouldn’t have to think about file types when the computer can figure it out for you.
Configuration Contexts: Multi-Tenancy Made Trivial
The global configuration pattern works beautifully for simple applications. But the moment you need different configurations for different customers, environments, or features, that simplicity becomes a liability.
We could have forced everyone to pass configuration objects around. We could have built some complex dependency injection system. Instead, we built contexts:
# Each tenant gets their own isolated configuration
tenant_context = RubyLLM.context do |config|
config.openai_api_key = tenant.openai_key
config.anthropic_api_key = tenant.anthropic_key
config.request_timeout = 180 # This tenant needs more time
end
# Use it without polluting the global namespace
response = tenant_context.chat.ask("Process this customer request...")
# Global configuration remains untouched
RubyLLM.chat.ask("This still uses your default settings")
Simple, elegant, Ruby-like. Your multi-tenant application doesn’t need architectural gymnastics. Each context is isolated, thread-safe, and garbage-collected when you’re done with it.
Perfect for multi-tenancy, A/B testing different providers, environment targeting, or any situation where you need temporary configuration changes.
Local Models with Ollama
Your development machine shouldn’t need to phone home to OpenAI every time you want to test something:
RubyLLM.configure do |config|
config.ollama_api_base = 'http://localhost:11434/v1'
end
# Same API, different model
chat = RubyLLM.chat(model: 'mistral', provider: 'ollama')
response = chat.ask("Explain Ruby's eigenclass")
Perfect for privacy-sensitive applications, offline development, or just experimenting with local models. This matters for development, for testing, for compliance, for costs. Sometimes the best model is the one running on your own hardware.
Hundreds of Models via OpenRouter
Access models from dozens of providers through a single API:
RubyLLM.configure do |config|
config.openrouter_api_key = ENV['OPENROUTER_API_KEY']
end
# Access any model through OpenRouter
chat = RubyLLM.chat(model: 'anthropic/claude-3.5-sonnet', provider: 'openrouter')
One API key, hundreds of models. Simple.
The End of Manual Model Tracking
Here’s where things get revolutionary. We’ve partnered with Parsera to create a single source of truth for LLM capabilities and pricing. When you run RubyLLM.models.refresh!
, you’re now pulling from the Parsera API - a continuously updated registry that scrapes model information directly from provider documentation.
No more manually updating capabilities files every time OpenAI changes their pricing. No more hunting through documentation to find context windows. Context windows, pricing, capabilities, supported modalities - it’s all there, always current.
However, providers don’t always document everything perfectly. We discovered plenty of older models still available through their APIs but missing from official docs. That’s why we kept our capabilities.rb
files - they fill in the gaps for models the Parsera API doesn’t cover yet. Between the two sources, we support virtually every model worth using.
Read more about this revolution in my previous blog post.
Rails Integration That Finally Feels Like Rails
The Rails integration now works seamlessly with ActiveStorage:
# Enable attachment support in your Message model
class Message < ApplicationRecord
acts_as_message
has_many_attached :attachments # Add this line
end
# Handle file uploads directly from forms
chat_record.ask("Analyze this upload", with: params[:uploaded_file])
# Work with existing ActiveStorage attachments
chat_record.ask("What's in my document?", with: user.profile_document)
# Process multiple uploads at once
chat_record.ask("Review these files", with: params[:files])
We’ve brought the Rails attachment handling to complete parity with the plain Ruby implementation. No more “it works in Ruby but not in Rails” friction.
Fine-Tuned Embeddings
Custom embedding dimensions let you optimize for your specific use case:
# Generate compact embeddings for memory-constrained environments
embedding = RubyLLM.embed(
"Ruby is a programmer's best friend",
model: "text-embedding-3-small",
dimensions: 512 # Instead of the default 1536
)
Enterprise OpenAI Support
Organization and project IDs are now supported for enterprise deployments:
RubyLLM.configure do |config|
config.openai_api_key = ENV['OPENAI_API_KEY']
config.openai_organization_id = ENV['OPENAI_ORG_ID']
config.openai_project_id = ENV['OPENAI_PROJECT_ID']
end
Rock-Solid Foundation
We now officially support and test against:
- Ruby 3.1 to 3.4
- Rails 7.1 to 8.0
Your favorite Ruby version is covered.
Ship It
gem 'ruby_llm', '1.3.0'
As always, we’ve maintained full backward compatibility. Your existing code continues to work exactly as before, but now with magical attachment handling and powerful new capabilities.
A Growing Community
This release includes contributions from 13 new contributors, with merged PRs covering everything from foreign key improvements to HTTP proxy support. The Ruby community continues to amaze me with its thoughtfulness and attention to detail.
Special thanks to @papgmez, @timaro, @rhys117, @bborn, @xymbol, @roelbondoc, @max-power, @itstheraj, @stadia, @tpaulshippy, @Sami-Tanquary, and @seemiller for making this release possible.
This Is Just The Beginning
Want to shape RubyLLM’s future? Join us on GitHub.
The future of AI development in Ruby has never been brighter. ✨